For security reason sometime we need password locking controller. In This article we developed a password locker. Since password is nothing but a character of string. So we need to control string function carefully. Let’s begin our journey with string handling.
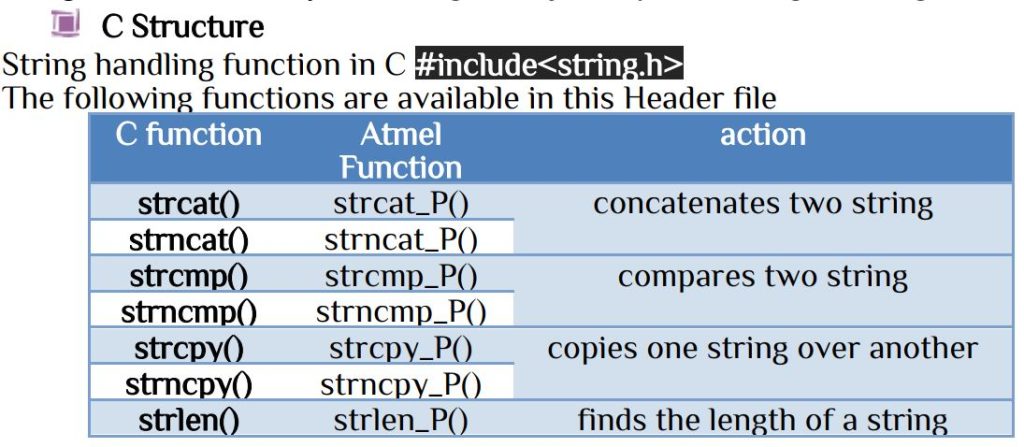
strcat() and strncat()
The strcat() or strncat() function join two strings together.
strcat(string1,string2);
Here all the characters of string2 are added to string1 by removing the null character at the end of string1.
strncat(string1,string2,n); here n is the number of characters
This statement will concatenate the left-most n characters of string2 and add to string1 by removing the null character at the end of string1.
strncmp() and strncmp()
The strcmp() function compares two string indentified by the arguments and has a value 0 if they are equal. If they are not equal, it contents the numeric difference between the first no matching characters in the strings.
i.e. int i;
i=strcmp(string1,string2);
Similarly, strncmp(string1,string2); compares the left most n characters of string1 to string2 and returns
- 0 if they are equal
- negative number, if string1 sub-string is less than string2 and
- positive number, otherwise
strcpy() and strncpy()
strcpy(string1,string2);
This statement copies the characters of string2 to string1.
strncpy(string1,string2); here n is the number of characters
This statement copies the first n characters of the source string2 into the target string1.
strlen() function
This function returns the number of characters in a string.
i.e. int n;
n= strlen(string); Let make a password locker with 3×4 matrix keypad display in which a predefined password is stored in the flash memory and as soon as the password match, a bit active. In this program we give 4 chances to put the right password. If the password is correct than PINC0 will be set to active some relay. If the password is incorrect in four chances then the processor will blocked and no effect on the key-button.
#include<avr/eeprom.h>
#include<avr/io.h>
#include<stdio.h>
#include<string.h>
#include<util/delay.h>
#include"keypad.h"
#include"lcd.h"
unsigned char j,display[20];
char StringData[]="pass091109"; /*****This is your passward 091109***********/
char im[20]="pass";
uint8_t sample,chance=4;
int locker;
int main(void)
{
/********************************Store in EEPROM*****************************************/
eeprom_update_block (( const void *) StringData , ( void *) 12, sizeof(StringData));
/****************************************************************************************/
keypad_init();
DDRC|=(1<<DDC0);
LCD_INIT();
DDRC&=~(1<<DDC1); //Enter key
PORTC|=(1<<PC1);
START:;
LCD_Clear();
LCD_write_string(1,1,"Press any key");
_delay_ms(1000);
sample=PINC&(1<<PC1); //PulUp internal resistance
while(sample)
{
sample=PINC&(1<<PC1);
j=key_press(0x7f);
LCD_write_string(number,2,"*");
im[number+3]=j;
chance=chance-(!sample);
}
/*Filter section*/
/**************************************************************************************/
im[3]='s';
char StringOfData [sizeof(StringData)];
eeprom_read_block (( void *) StringOfData , ( const void *) 12, sizeof(StringData)) ;
/**************************************************************************************/
locker=strcmp(StringOfData,im);
if(locker)
{
PORTC&=~(1<<PC0);
LCD_write_string(1,1,"Pass. incurrect");
cleardata(); //clear storage
_delay_ms(1000);
sprintf(display,"%d chance left ",chance);
LCD_write_string(1,1,display);
_delay_ms(1000);
if(chance==0)
{
LCD_write_string(1,1,"****************");
LCD_write_string(1,2,"*****locked*****");
goto END;
}
goto START;
}
else
{
PORTC|=(1<<PC0);
LCD_write_string(1,1,"Passward currect");
LCD_write_string(1,2,"******open******");
}
END:;
return 0;
}
Here the default password is —— 091109
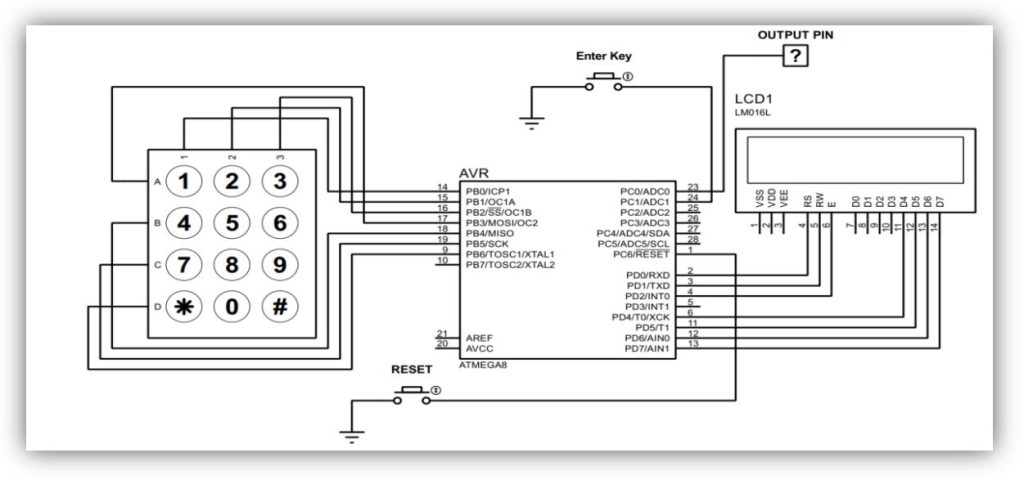